If you have worked with Java, getting started with Kotlin is very easy, because Kotlin was born to improve the unnecessary things of Java, helping us focus on the business of the application instead of having to write a lot of lines of code.
For example, for a Hello World application with the main() method to print the text “Hello World”:
- With Java we need to create a new .java file, then define the class that comes with this .java file. Next, create a new main() method to use System.out.println() to print the text “Hello World”:
1 2 3 4 5 6 7 |
package com.huongdankotlin; public class HelloWorld { public static void main(String[] args) { System.out.println("Hello World"); } } |
- With Kotlin, you also need to create a new .kt file, but you don’t need to define a class name. You can write the static main() method directly to print the word “Hello World”:
1 2 3 4 5 |
package com.huongdankotlin fun main(args: Array<String>) { println("Hello World") } |
You don’t even need a semicolon at the end!
Here, as you can see in the above example, the method in Kotlin will start with the keyword “fun”. Method parameters start with the parameter name, then the data type of this parameter. The parameter names and data types are separated by a colon “:”.
You can remove the args parameter in the main() method above because in the body of the method, we are not using this parameter:
1 2 3 4 5 |
package com.huongdankotlin fun main() { println("Hello World") } |
The result is still the same:
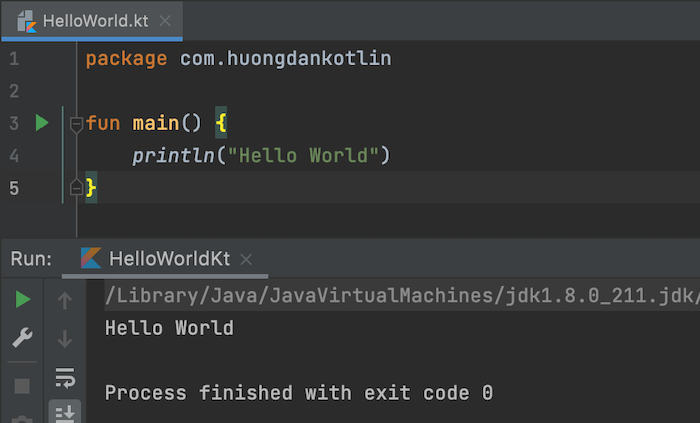
Kotlin uses wrappers for common code in Java. In the above example to print the text “Hello World”, as you can see, instead of writing:
1 |
System.out.println("Hello World"); |
in Java, now we just need to write:
1 |
println("Hello World") |
in Kotlin.
If your method returns a result, then in Kotlin, the way it is written is also different.
We will put the return data type right after the method and parameter name declarations, separated by a colon, as follows:
1 2 3 |
fun getName(): String { return "Khanh"; } |
Example:
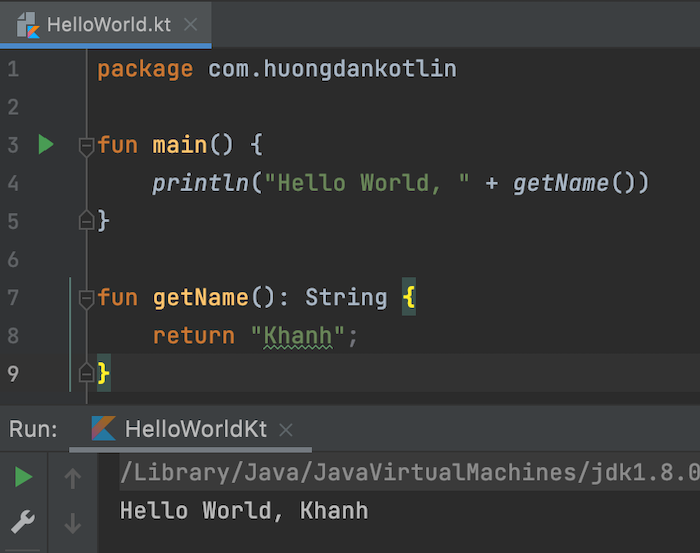
In Kotlin, we don’t use the new operator to initialize an object.
Kotlin omits this operator, but we can also instantiate an object, like this:
1 2 3 4 5 6 7 8 |
package com.huongdankotlin fun main() { val student = Student() student.name = "Khanh" println(student.name) } |
The Student class has the following content:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
package com.huongdankotlin; public class Student { private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } } |
We will declare the variable starting with the val keyword and with the Getter or Setter method, we do not need to call these methods directly, just call the variable name with the property. Actually, Kotlin will also compile bytecode like Java but just omitted for brevity! The result is the same as we do in Java, as follows:
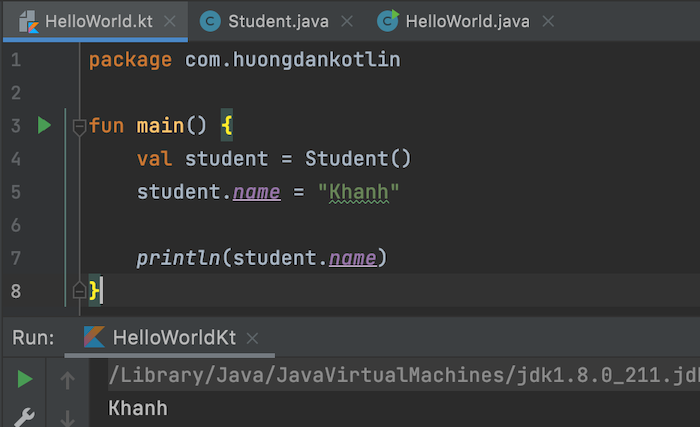
In Kotlin we can use wrapper arrayListOf() to instantiate an ArrayList object.
Accessing the elements in this ArrayList is also much easier:
1 2 3 4 5 6 7 |
package com.huongdankotlin fun main() { val students = arrayListOf("Khanh", "Huong Dan Java") println(students[1]) } |
Result:
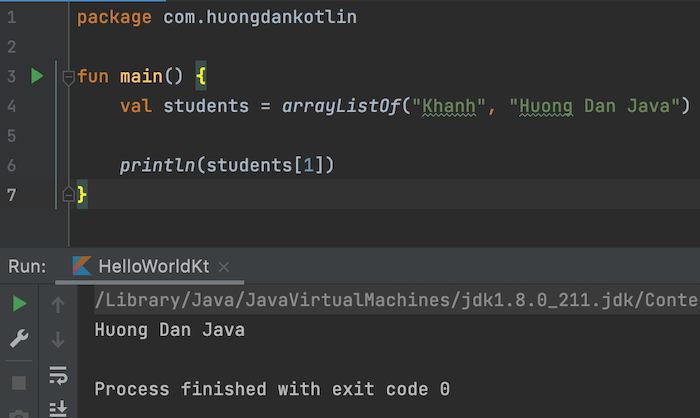
As you can see, we also do not need to use the get() method to access the elements in the List. Just use square brackets and specify the index of the element to access.
For Exception, in Kotlin, we don’t have a distinction between Checked Exception and Runtime Exception.
All exceptions in Kotlin are Runtime Exceptions. If in Java, the following code, you have to handle the exception:
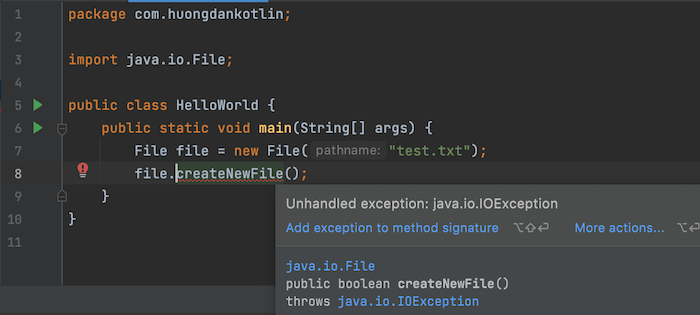
In Kotlin, you don’t need to do this:
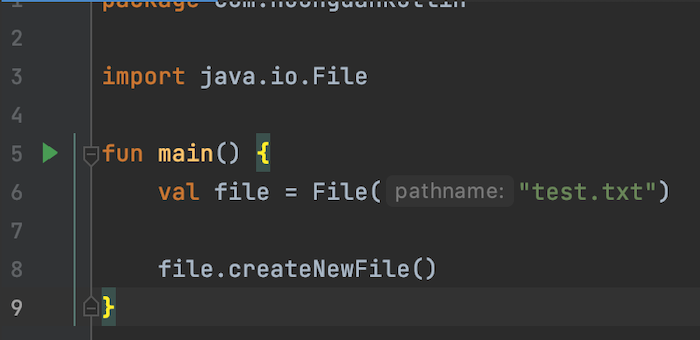
Of course, you can also use try … catch to handle exceptions. But not required at compile time.
There are many more differences between Java and Kotlin. Please find out more!